Android App Setup
The Button Merchant Library for Android is a lightweight, open-source library designed to manage the Button Attribution Token. By the end of this section, you’ll be able to associate all incoming users against their subsequent taps in other applications that brought them to your Android application.
Add the Merchant Library
Include Dependency
To get started, add the Button Merchant Library to your build.gradle
file in the dependencies.
implementation 'com.usebutton.merchant:button-merchant:1+'
Allow Gradle to download and include the Button Merchant Library before continuing.
Setup Library
Next, import and configure the Button Merchant Library in either the Application
subclass (preferred), or in the launching Activity
.
import com.usebutton.merchant.ButtonMerchant
class MyApplication : Application() {
override fun onCreate() {
super.onCreate()
// Replace app-xxxxxxxxxxxxxxxx with your App ID from the
// Button Dashboard: https://app.usebutton.com
ButtonMerchant.configure(this, "app-xxxxxxxxxxxxxxxx")
}
}
import com.usebutton.merchant.ButtonMerchant;
public class MyApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
// Replace app-xxxxxxxxxxxxxxxx with your App ID from the
// Button Dashboard: https://app.usebutton.com
ButtonMerchant.configure(this, "app-xxxxxxxxxxxxxxxx");
}
}
Note
The Button Merchant Library is disabled by default. No user activity is reported and no data is collected until configured with an application id. Initialization can be deferred by simply not calling this method until ready to do so.
Additionally, some users will be initializing your application from a fresh install after following a promotion. In order to fulfill these specific user requests, include a call from within your application’s main Activity
onCreate
method to ButtonMerchant.handlePostInstallIntent
. This will fetch the user’s intent prior to the fresh install, and route them directly to your relevant promotion. The handlePostInstallIntent
method will pass a non-null url to the listener only once, upon first app launch following an install from a Button Link.
import com.usebutton.merchant.ButtonMerchant
class MainActivity : Activity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
ButtonMerchant.handlePostInstallIntent(this) { intent, throwable ->
// If Intent is available, start it
intent?.let { startActivity(it) }
// Or if an error is provided, handle that
throwable?.let {
Log.e("Button", "Error checking post install Intent", it)
}
}
}
}
import com.usebutton.merchant.ButtonMerchant;
public class MainActivity extends Activity {
@Override
public void onCreate() {
super.onCreate();
ButtonMerchant.handlePostInstallIntent(this, new PostInstallIntentListener() {
@Override
public void onResult(@Nullable Intent intent, @Nullable Throwable t) {
if (intent != null) {
startActivity(intent);
} else if (t != null) {
Log.e(TAG, "Error checking post install Intent", t);
}
}
});
}
}
Proguard Rules
If your app is using Proguard, make sure it includes rules for the Button Merchant Library. This file is usually located in yourapp/proguard-rules.pro
, or its location is specified in build.gradle
, look for proguardFiles
in your default or variant configuration (note: this can be multiple files).
Here is an example proguard-rules.pro
snippet including the Button Merchant Library
-keepattributes Exceptions,InnerClasses,EnclosingMethod
-keep class com.usebutton.** { *; }
-keepclassmembers class * implements android.os.Parcelable {
static ** CREATOR;
}
-keep class com.google.android.gms.ads.identifier.AdvertisingIdClient { public *; }
Register Deep Links
Button’s Merchant Library will abstract away any complexities of token management for you, including extracting the token from any incoming deeplinks.
For users that have already installed your application and are entering from another application’s promotion, pass the incoming URL to the Button Merchant Library in order to accurately attribute and handle their intent.
The ButtonMerchant
class allows you to handle both Android App Links and Custom Scheme URLs by passing the user intent to trackIncomingIntent
.
This method should be called from the onCreate
method in your launch Activity
or whichever Activity
handles incoming links. For example, if you use Verified App Links to directly open the product Activity
, ButtonMerchant.trackIncomingIntent
should be called from ProductActivity.onCreate
.
It is important that trackIncomingIntent
is called first in the Activity
that handles incoming links. If the link that opens the app is not a Button Link, the Merchant Library will make this determination and Button will not be a part of the user journey.
import com.usebutton.merchant.ButtonMerchant
class ProductActivity : Activity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Handle custom scheme deeplinks and App Links
ButtonMerchant.trackIncomingIntent(this, intent)
}
}
import com.usebutton.merchant.ButtonMerchant;
public class ProductActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Handle custom scheme deeplinks and App Links
ButtonMerchant.trackIncomingIntent(this, getIntent());
}
}
Note
If your Activity is set to run in a non-standard
launchMode
such assingleTop
,singleTask
, orsingleInstance
mode, please make sure to callButtonMerchant.trackIncomingIntent(~)
from theonNewIntent
method in addition to the aforementionedonCreate
.
Setup your Button Links domain
Once you’ve registered your Button Links subdomain (yourdomain.bttn.io
), the last step is to add support for those links via intent filter within your Activity Declaration. If you’ve already registered an intent filter for your domain, you can simply add another to the same Activity Declaration.
We support verified web associated App Links so make sure to add the autoVerify="true"
attribute to your intent filter.
Note
This support is part of Android Marshmallow (6.0), so you'll also need to build against this target Button Merchant Library level.
<activity name=".MainActivity">
<!-- Make sure to add this as a NEW Intent Filter in your Activity block -->
<intent-filter android:autoVerify="true">
<action android:name="android.intent.action.VIEW"/>
<category android:name="android.intent.category.DEFAULT"/>
<category android:name="android.intent.category.BROWSABLE"/>
<data
android:host="yourdomain.bttn.io"
android:pathPattern=".*"
android:scheme="https" />
</intent-filter>
</activity>
Button hosts the Digital Asset Links JSON file for your bttn.io
subdomain.
-
Once you have your updated Android Universal Link, navigate to the Button Dashboard Apps Section to add your certificate fingerprint.
-
Choose your Android application and click its name to drill in.
-
Scroll down to the Configurations section and select Add Manual Configuration. If you've already added one, click Edit on the right side of that record.
-
You can add your package name and sha256 certificate fingerprints to your app configuration here.
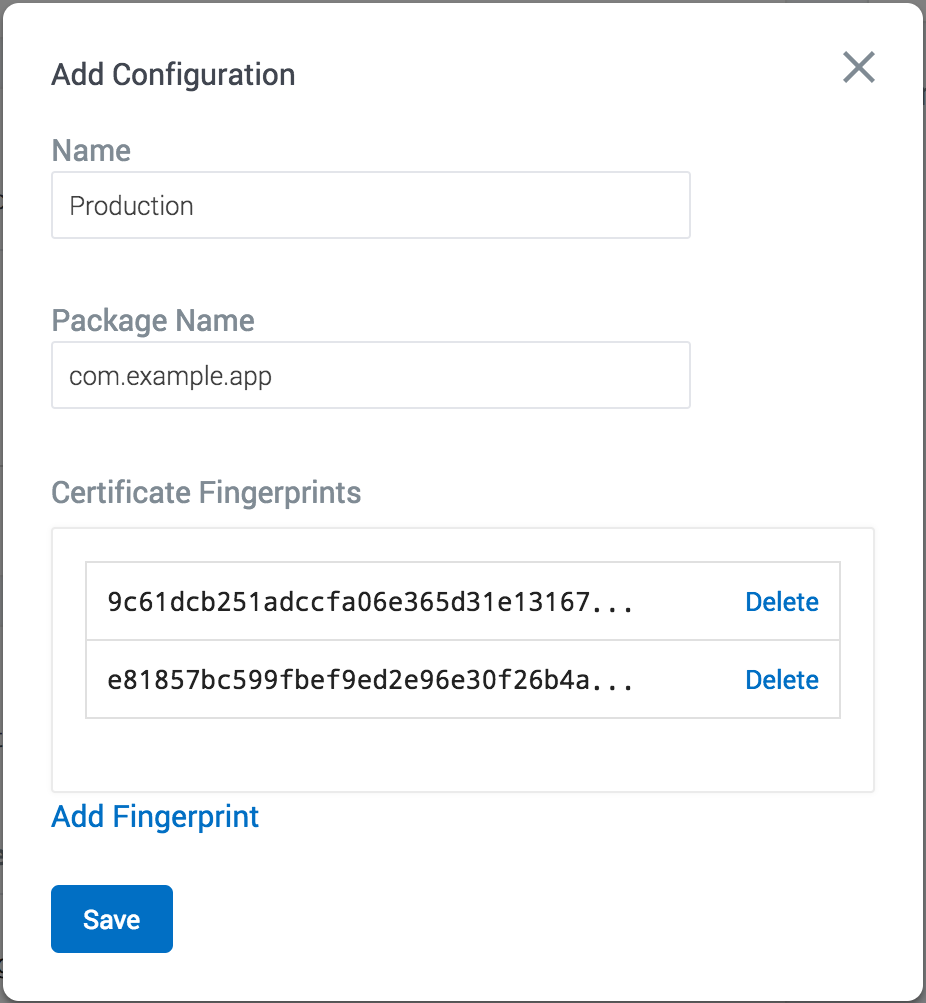
Use the following command to generate the fingerprint via the Java keytool:
keytool -list -printcert -jarfile <APK_FILE> | grep SHA256
Now, your link should open your app without ever opening your browser when the app is installed.
Note
By supporting web associated app links you will prevent the Intent Chooser when opening the URL and your app will open automatically.
Updated 9 days ago